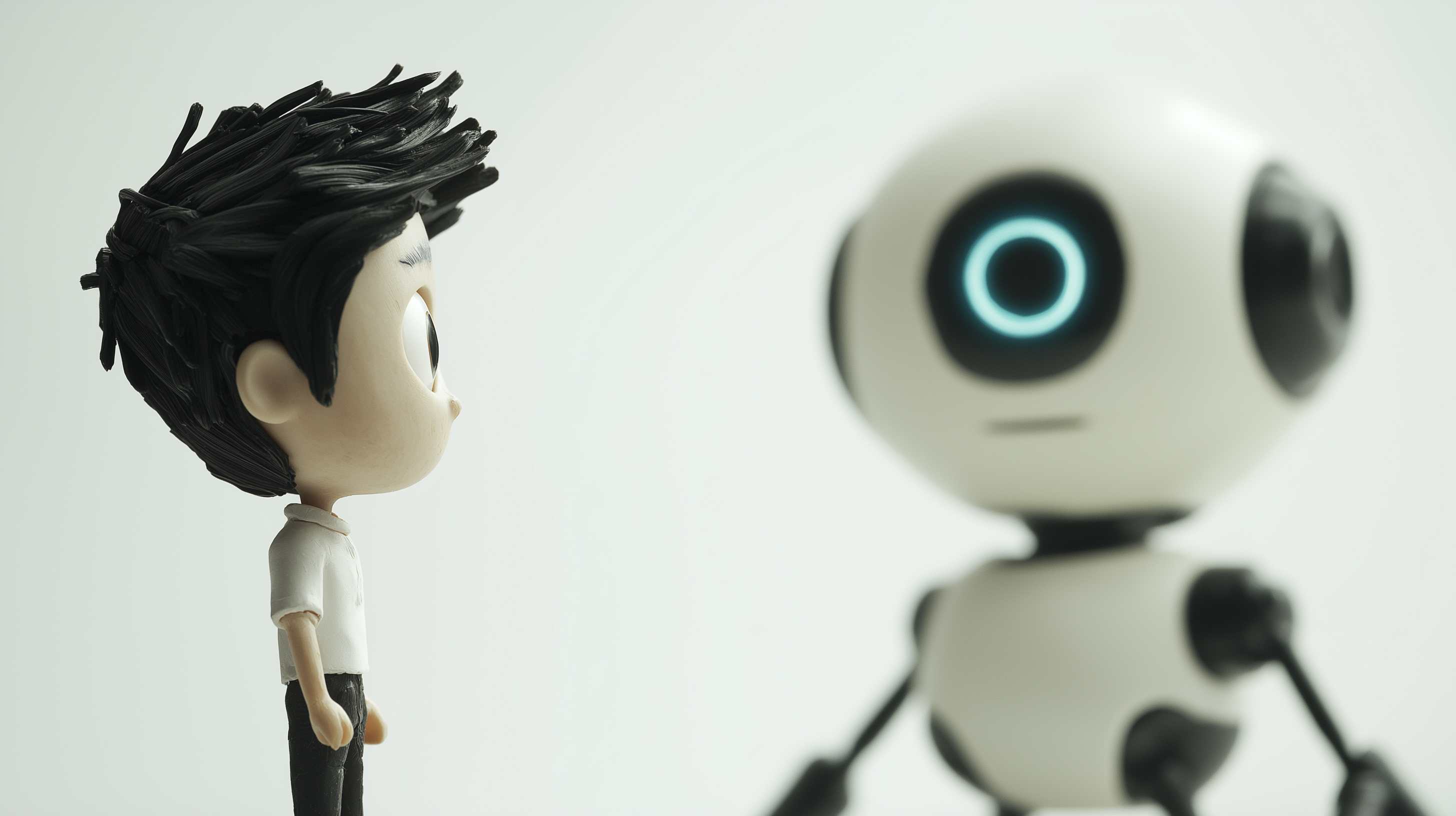
Modern Java Development Best Practices in 2023
Explore the latest best practices for Java development including functional programming patterns, new language features, and performance optimizations.
Modern Java Development Best Practices in 2023
Java has evolved significantly since its inception, and staying current with modern practices is essential for writing clean, efficient, and maintainable code. In this first article of our Java Mastery series, we’ll explore the most important best practices for Java development in 2023.
Embrace New Language Features
Modern Java offers numerous features that make your code more concise and readable:
// Old way - verbose and error-prone
List<String> names = new ArrayList<>();
names.add("Alice");
names.add("Bob");
names.add("Charlie");
// Modern way with factory methods
var names = List.of("Alice", "Bob", "Charlie");
// Old way - verbose null check
String value = getStringValue();
if (value != null) {
processString(value);
}
// Modern way with Optional
Optional.ofNullable(getStringValue())
.ifPresent(this::processString);
Adopt Functional Programming Concepts
Functional programming paradigms can significantly improve code quality:
// Imperative approach
List<Integer> numbers = Arrays.asList(1, 2, 3, 4, 5);
List<Integer> doubled = new ArrayList<>();
for (Integer n : numbers) {
doubled.add(n * 2);
}
// Functional approach
List<Integer> doubled = numbers.stream()
.map(n -> n * 2)
.collect(Collectors.toList());
// Using method references
List<String> names = Arrays.asList("Alice", "Bob", "Charlie");
names.forEach(System.out::println);
Use Modern Tools and Frameworks
The Java ecosystem offers excellent tools for development:
- Build Tools: Gradle or Maven for dependency management
- Testing: JUnit 5, AssertJ, and Mockito
- Monitoring: Micrometer with Prometheus
- Containers: Docker and Kubernetes for deployment
- Cloud: Spring Cloud for cloud-native applications
Follow Architecture Patterns
Modern Java applications benefit from these architectural patterns:
- Hexagonal Architecture: Separate business logic from external concerns
- Microservices: Build small, focused services (when appropriate)
- Event-Driven Architecture: Use events for communication between components
- Domain-Driven Design: Model software based on the domain
Security Best Practices
Security is paramount in modern applications:
// Avoid using outdated security practices
String password = "password123"; // DON'T store plaintext!
// Better approach with modern hashing
String hashedPassword = BCrypt.hashpw(password, BCrypt.gensalt());
// Input validation is essential
public void processUserInput(String input) {
// Validate input before processing
if (!InputValidator.isValid(input)) {
throw new IllegalArgumentException("Invalid input");
}
// Process valid input
}
Performance Considerations
Performance optimization remains important:
- Use proper data structures for your use case
- Lazy initialization for expensive objects
- Concurrent collections for multi-threaded code
- Avoid premature optimization - measure first!
- Consider JVM options for better garbage collection
Conclusion
Java continues to evolve, and so should your coding practices. By adopting these modern approaches, you’ll write more maintainable, secure, and efficient code.
Stay tuned for the next article in our Java Mastery series, where we’ll dive deeper into functional programming patterns in Java!
More in this series
View full seriesJava Mastery Series
You're reading part 1 of this series